A stack is a LIFO
(Last In First Out) Data structure - like a stack of plates being
washed - a new plate gets placed on the top of the stack, and if
one is removed, it is also removed from the top.
Adding a new element to a stack is called pushing... ...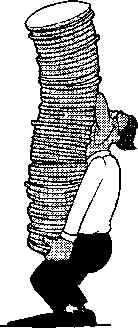
......removing
one is called popping.
With every stack there is a stack pointer showing where the top of the stack is.
(When
implementing a stack in a high level language you will need an array to hold the elements of the stack and an integer
variable 'TopOfStack')
Example : The diagram shows a stack with three names
already in it. :
8 |
|
STACK
POINTER = 3 |
7 |
|
6 |
|
5 |
|
4 |
|
3 |
TODDS |
2 |
JONES |
1 |
GUDRUNSON |
If a new name 'EGBERT' is added to (pushed
onto) the stack, the new stack looks like......
8 |
|
STACK
POINTER =4 |
7 |
|
6 |
|
5 |
|
4 |
EGBERT |
3 |
TODDS |
2 |
JONES |
1 |
GUDRUNSON |
(Note that the Stack Pointer
has been incremented).
Stacks are very useful data
structures in computing.. Uses include:
- Storing return
addresses. When a
program is run and a subroutine is called, the address
where the processing stopped is pushed onto a stack. The
subroutine is run, and when done, the processing returns
to the address on the top of the stack (which is popped
off). This means that subroutines may call subroutines
etc..
- Stacks are also used to evaluate
arithmetical expressions given in Reverse Polish
Notation
|